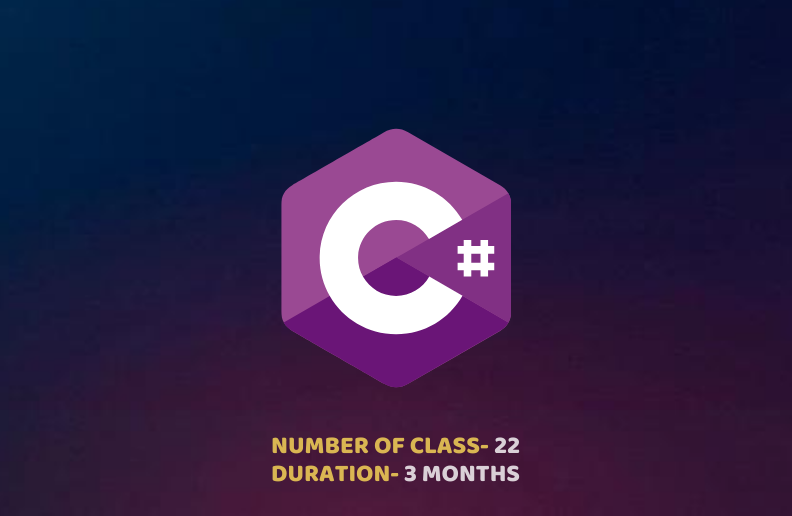
Who Can Join This Course?
Fluent knowledge of C/C++ or any other basic programming language
Course Outline
Getting Started.
● Course Rules.
● Introduction to slack channel.
● Introduction to tracker.
● Introduction to Visual Studio.
● Creating first console project.
Version controlling using git.
● Importance of version control.
● One step VS two step version control.
● Understanding repository.
● Basic git operations.
● Using git using Https & SSH.
● Creating and merging branches.
● Conflict resolution.
Working with basic data types.
● C# data types.
● Variables & constants.
● Array, Multidimensional Array, Jagged Array.
● Input / Output processing.
Statements & Operators.
● Conditional Statements.
● Arithmetic Operators.
● Bitwise Operators.
● Logical Operators.
● Loops.
Operations With Text.
● Important String methods.
● String formatting.
● Character methods.
Working with Class
● Class.
● Namespaces.
● Field, properties.
● Access Modifiers.
● Auto properties.
● Method Overloading.
● Constructor, Destructor/Finalizer.
● Constructor chaining.
● Readonly, const keywords.
Working More with Classes.
● Static Class.
● Abstract Class.
● Interface.
● Method Override, virtual, new, sealed keywords.
● Partial Class.
Other Types.
● Structure.
● Enum.
● DateTime.
● StringBuilder.
● Parameter Modifier.
● Tuple.
● Nullable type.
● Anonymous object.
● dynamic keyword.
Using Generics.
● Generic Class.
● Generic Method.
● Constraints.
● Default keyword.
● Built in Generic data structures.
● Non-generics collection.
Working with Data Access.
● ADO.NET intro.
● CRUD operations.
● SQL Server basics.
Working with Entity Framework.
● DbContext configuration.
● Entity relationship using fluent API.
● Migrations.
● Data Annotations.
File & Streams
● Use of FileInfo & File
● Use of DirectoryInfo & Directory
● File read & write operations
● Use of Streams
● Use of “using” keyword
Important Interfaces.
● IEnumerable.
● ICloneable.
● IDisposable.
● ICollection.
Learn OOP Principles.
● Encapsulation.
● Inheritance.
● Abstraction.
● Polymorphism.
Learn SOLID Principles.
● Single Responsibility Principle.
● Open Closed Principle.
● Liskov Substitution Principle.
● Interface Segregation Principle.
● Dependency Inversion Principle.
C# Advance Features.
● Delegates.
● Events.
● Func & Action.
● Lambda method.
● Linq.
More Advanced Features.
● Threading.
● Async.
● Exception Handling.
● Indexers.
● Reflection.
● Extension method.
● Expression.
New Features in C#.
● New switch statements.
● Interface default implementation.
● Inverse indexing.
● Records.
Batch 19
Schedule
Starts from Dec 02, 2024
Last date of registration is Nov 14, 2024 (16 Days Remaining)
Register Now Pay NowInstructors

I am founder & CEO of Dev Skill. I am also a software architect, passionate trainer and consultant.
LinkedIn ProfileWhat Students Said
I recently completed the C# course under Jalal Uddin Sir, and it was an excellent experience. The course materials were well-organized, and the classes were enjoyable and informative. The topics were delivered in a clear and easy-to-understand manner, with professional explanations that made everything accessible. The question-answer sessions were particularly helpful, as they addressed any doubts from previous lessons. The exams, though a bit challenging, required a solid understanding of the core concepts, which I appreciated. Overall, I highly recommend this course to newcomers.

Professional Programming with C# Batch: 17
After completion of the course Professional Programming with C#, I have gained my confidence in programming. The course content is incredibly rich, covering all key areas of programming. And then the trainer's deep knowledge and passion for the subject were evident from the start. He is not only proficient in the technical aspects of C# but also excellent at breaking down complex concepts into digestible lessons that catered to all learning levels. His dedication, patience, and hands-on approach helped me build a solid foundation in C# and grow my confidence as a programmer. I highly recommend Dev Skill to anyone looking to improve their programming skills.
Professional Programming with C# Batch: 17
All praise belongs to Allah, who has granted me the opportunity to embark on this incredible learning journey. This course, under the guidance of Jalal Uddin Sir, has truly been a transformative experience. The course is not only well-structured but also highly engaging, making it suitable for both beginners and more experienced learners. One of the standout aspects of this course is the way Sir teaches. His clarity, patience, and passion for programming make even the most complex topics seem approachable. His advice throughout the course pushed me to continuously improve and stay focused. I especially appreciate the Q&A sessions, where Sir takes the time to address every query thoughtfully, ensuring no one is left behind. The assignments, though challenging, have been a key factor in my learning process. I am truly grateful to Jalal Uddin Sir for his dedication and for creating such a positive and supportive learning environment. I highly recommend this course to others.
Professional Programming with C# Batch: 17
I really liked how the course was structured; it was clear and to the point, which made it easy to follow. The classes were informative, and the assignments were helpful in practicing what we learned. I also really appreciate the effort the instructor put into making everything engaging and easy to understand. If anything, more feedback on the assignments would be great!
Professional Programming with C# Batch: 17
I recently completed the "Professional Programming with C#" course through Dev Skill. The course provided a solid foundation in OOP, advanced C# features, Git, and CRUD operations. Jalal Uddin Sir’s insights and best practices were precious. To further improve the learning experience, I suggest adding detailed explanations for each topic and including more practice problems. A dedicated website providing these resources would offer a more structured way for learners to engage with the course content and enhance their understanding.

Professional Programming with C# Batch: 17