Data Structure & Algorithm Fundamentals
Data Structure & Algorithm Fundamentals
Beginner
Tk. 5,000
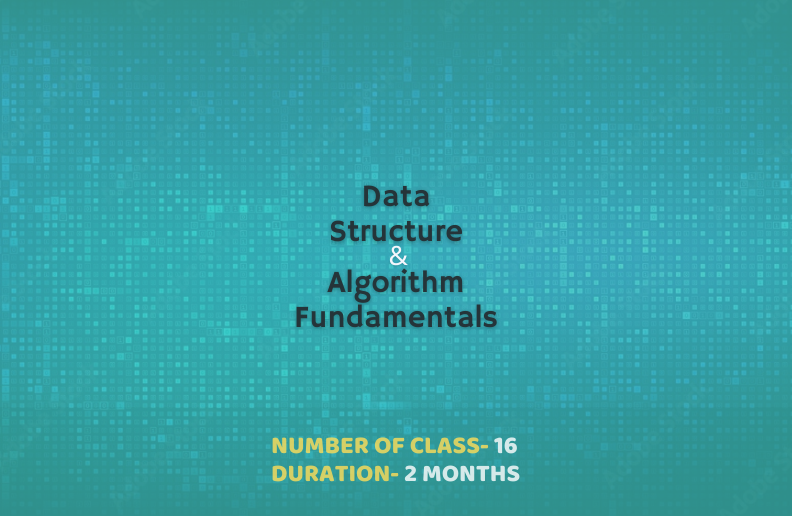
Who Can Join This Course?
Basic knowledge (i.e. loops, arrays, I/O etc) of at least one programming language.
Course Outline
Complexity Analysis.
● Time Complexity Analysis.
a. Big-O Notation.
b. How to calculate time complexity of a function.
● Memory Complexity Analysis.
a. Capacity of each data type variable and its calculation.
b. How to calculate memory complexity of a piece of code.
c. Concepts of Heap Memory & Stack Memory.
● Single and Multidimensional arrays.
a. Introduction.
b. Conversion between various dimensional arrays into 1D arrays.
c. Application and necessity of arrays.
Sorting Algorithms.
● Introduction.
● Implementation in C++.
● Complexity analysis of sorting algorithms.
Greedy Algorithms.
● Introduction.
● Discussion on a few related problems.
● Fractional Knapsack, etc.
Searching Algorithms. Part-1
● Binary Search.
a. Introduction.
b. Implementation in C++.
c. Different application of searching techniques.
Part-2
● Ternary Search
a. Introduction.
b. Implementation in C++.
c. Different application of searching techniques.
Linked List.
● Introduction.
● Why we need it.
● How it works.
● Implementation in C++.
● Implementing with static array and dynamic array.
Stack.
● Introduction, why we need it, how it works.
● Implementation in C++ with static array, dynamic array and linked list.
● Different usage of Stack.
Queue.
● Introduction, why we need it, how it works.
● Implementation in C++ with static array, dynamic array and linked list.
● Different usage of queue.
Introduction to Graph Theory.
● Introduction.
a. What is a graph?
b. Why do we need this?
● Types of graph.
a. Directed Graph and Undirected Graph.
b. Cyclic.
c. Tree.
Graph Representation.
● Graph modeling.
● Adjacency matrix representation.
● Adjacency list representation.
● Edge List representation.
● Implementing all the representations in C++.
Recursion.
● Intro to recursion and backtracking.
● Relevant basic problem solving i.e. 8-queen, all permutations, Fibonacci etc.
Sorting Algorithm.
● Merge Sort.
a. Introduction.
b. Implementation in C++.
● Quick Sort.
a. Introduction.
b. Implementation in C++.
Greedy Algorithm.
● Minimum Spanning Tree.
a. Introduction.
b. Discussion on a few related problems.
Graph Traversing Algorithm.
● Depth First Search.
a. Introduction.
b. Implementation in C++.
Shortest Path Traversing Algorithm.
● Breadth First Search.
a. Introduction.
b. Find shortest path.
c. Implementation in C++.
● How to model a graph based on real life scenario and finding shortest.
● Different application of DFS and BFS other than graph.
Technical Assignments.
●There will be a set of technical assignments after completion of each module.
Instructors

I am an ICPC World Finalist 2024 with 8+ years of experience participating in many national & international contests. I am currently working as a Jr. Software Engineer at Kite Games Studio. My codeforces handle is MinhazIbnMizan.
LinkedIn ProfileWhat Students Said
I found this course very helpful. Sir has explained every class of the course very well. Class record is uploaded at the end of the class which is very helpful for me. Your data structure course is very helpful for my universities studies. I have benefited a lot of from this course
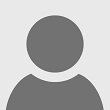
Data Structure & Algorithm Fundamentals Batch: 2
Its a highly recommended course for learning Basic Algorithm and Data Structure. Thorough discussion on each topic and answering topic related questions made it simple to understand.

Data Structure & Algorithm Fundamentals Batch: 1
This course has been helping me a lot to improve my Data Structure and Algorithm knowledge. Md Sadman Sakib vai is an amazing instructor.

Data Structure & Algorithm Fundamentals Batch: 1
This course is meant to be a starting point of problem solving world by learing entry level knowledge about data structure and algorithm. So this course helped me to make my footprint in problem solving. Though this course is not a C++ learing course and our teacher explicitely told us no need to worry about C++(even we present psudo code before him, students are welcomed), at least one class(C++ basics) would have been good for us who didn't code in C++. Overall I learned a lot from here and I have no complain. If I could not get benefit from this couse, I'm responsible for that by not practicing that much. I feel really grateful to course instructor Md Sadman Sakib bhai(many time I asked questions out of our syllabus and he answered very politely which is rere in Bangladesh).

Data Structure & Algorithm Fundamentals Batch: 1
ডাটা স্ট্রাকচার এবং এলগোরিদমের প্রথম কোর্সটি অত্যন্ত সুগঠিত এবং যথার্থ ছিল। কোর্সের ইন্সটাক্টর সাকিব ভাই আমাদেরকে প্রতিটি টপিক মার্জিত এবং সুনিপুণভাবে বুঝিয়েছিলেন যা আমাদেরকে কনসেপ্টগুলো পরিষ্কারভাবে বুঝতে সহায়তা করেছিল। আমরা কোর্স থেকে শুধু কোর্সের টপিক সংক্রান্ত লেখাপড়া করিনি বরং ভাই আমাদেরকে ডাটা স্ট্রাকচার এবং এলগোরিদমের প্রয়োগ নিয়ে সফ্টওয়্যার ইন্ডাস্ট্রিতে অনেক 'রিয়েল ফ্যাক্টগুলো' তুলে ধরেছিলেন এবং আমাদেরকে সেই অনুযায়ী প্রস্তুত হতে পরামর্শ দিয়েছিলেন যা অত্যন্ত উপকারী ছিল। আমি এই কোর্স থেকে অনেক উপকৃত হয়েছি এবং বিশেষত যারা সি এস ফিল্ডে বিগিনার আছেন, আমরা অনেকসময় কোর্সের উপর্যুক্ত টপিকগুলো নিয়ে হেলা-ফেলা করে থাকি। জ্ঞানের পরিধি অনুযায়ী, যদি নিজেকে আমরা এই সকল বিষয়ে যাচাই করতে চাই তাহলে আমার মতে এই কোর্সটি করে দেখতে পারেন এতে আপনাদের অনেক প্রশ্নের উত্তর নিহিত আছে। সর্বশেষে, ডেভস্কিল টিম এবং সাকিব ভাইকে ধন্যবাদ কোর্সটি সুসজ্জিতভাবে পরিচালনা করার জন্য।

Data Structure & Algorithm Fundamentals Batch: 1